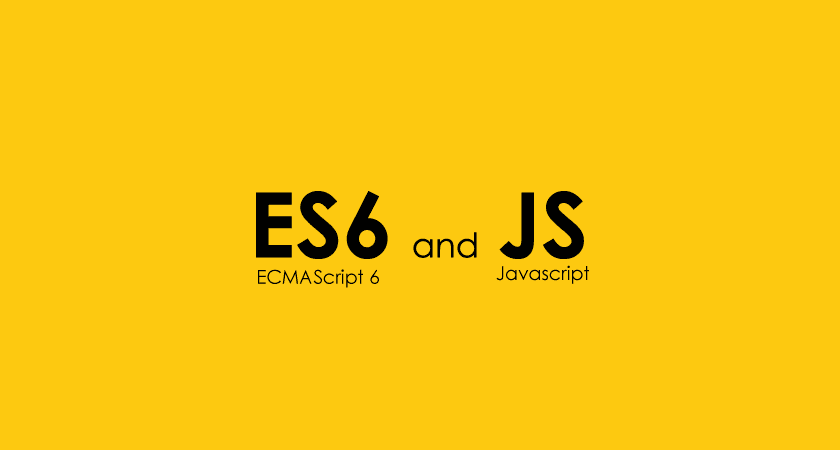
本文主要介绍,ES6新特性迭代器。迭代器(Iterator)是一种接口,为各种不同的数据结构提供统一的访问机制。任何数据结构只要部署Iterator接口(对象的一个属性),就可以完成遍历操作。
*** 使用场景: 需要自定义遍历数据的时候,我们可以使用迭代器。
ES6创建了一种新的遍历命令for...of循环,Iterator接口主要供for...of消费
// 声明一个数组
let arr = ['a','b','c','d'];
// 使用 for...of
for( let value of arr ){
console.log(value); // a,b,c,d
}
// 使用 for...in
for(let key in arr){
console.log(key); // 0,1,2,3
}
原生具备iterator接口的数据(可用for...of遍历)
* Array
* Arguments
* Set
* Map
* String
* TypedArray
* NodeList
工作原理
* 创建一个指针对象,指向当前数据结构的起始位置
* 第一次调用对象的next方法,指针自动指向数据结构的第一个成员
* 接来不断调用next方法,指针一直往后移动,直到指向最后一个成员
* 每调用next方法返回一个包含value和done属性的对象
// Symbol.iterator 工作原理
let iterator = arr[Symbol.iterator]();
// 调用对象的next方法
console.log(iterator.next()); // {value: 'a', done: false}
console.log(iterator.next()); // {value: 'b', done: false}
console.log(iterator.next()); // {value: 'c', done: false}
console.log(iterator.next()); // {value: 'd', done: false}
console.log(iterator.next()); // {value: undefined, done: true}
自定义遍历实例
// 自定义遍历对象里的data数据
const obj = {
name: "fujuhao.com",
data: ['a','b','c','d']
}
// 直接使用for...of遍历obj对象,提示:Uncaught TypeError: obj is not iterable
for(value of obj){
console.log(value);
}
// 这时我们可以给obj增加一个Symbol.iterator接口,达到按我们自己的需求来遍历出来
const obj2 = {
name: "fujuhao.com",
data: ['a','b','c','d'],
[Symbol.iterator]() {
// 索引变量
let index = 0;
return {
next: ()=>{
if(index < this.data.length){
const res = { value: this.data[index], done: false };
index++;
return res;
}else{
return { value: undefined, done: true };
}
}
}
}
}
// 打印出 a,b,c,d
for(value of obj2){
console.log(value)
}
下一篇:ES6新特性_生成器函数
The Posts
- Markdown与html互转markdown.js_showdown.js_marked.js_turndown.jsApr 5, 2022
- ES10新特性_fromEntries_trimStart_trimEnd_flat_flatMap_descriptionMar 24, 2022
- ES9_rest参数与es9_spread扩展运算符Mar 23, 2022
- ES6新特性_模块化(module)Mar 17, 2022
- ES6新特性_number数值扩展与object对象方法扩展Mar 16, 2022
- Angular12中使用wow.js页面滚动动画效果Mar 15, 2022
- ES6新特性_class类声明继承constructor,super,static,set,get详细介绍Mar 12, 2022
- ES6新特性_Map用法和使用场景size,get,set,has,clear,deleteMar 12, 2022
- ES6新特性_集合(set)Mar 10, 2022
- ES6新特性_Promise介绍与基本使用Mar 10, 2022
- ES6新特性_生成器函数Mar 9, 2022
- ES6新特性_迭代器Mar 9, 2022
- ES6新特性_Symbol基本使用Mar 9, 2022
- ES6新特性_扩展运算符Mar 9, 2022
- ES6新特性_rest参数Mar 8, 2022
- ES6新特性_函数参数的默认值Mar 8, 2022
- ES6新特性_箭头函数Mar 8, 2022
- ES6新特性_简化对象的写法Mar 8, 2022
- ES6新特性_模板字符串Mar 8, 2022
- ES6新特性_变量的解构赋值Mar 8, 2022