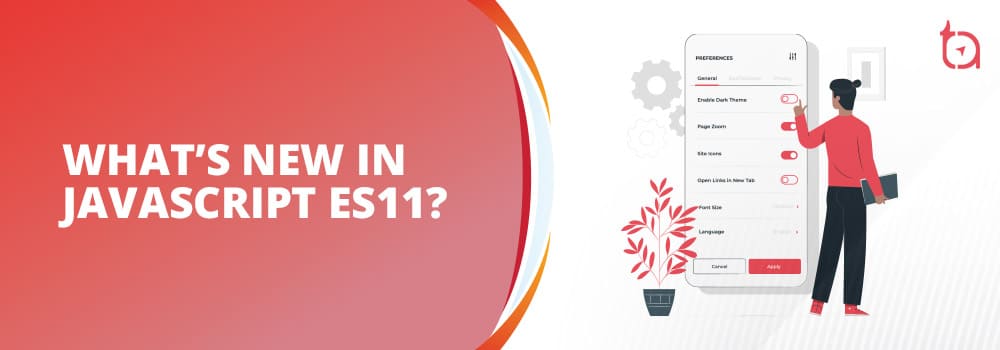
本文主要介绍,ES11新特性、matchAll、私有属性、allSettled、可选链操作符、动态import导入、BigInt 和 globalThis对象。
ES11新特性 1、String.prototype.matchAll
ES11的 matchAll 用来得到正则批量匹配的结果;
let str = `
<ul>
<li>
<a>name</a>
<p>https://www.fujuhao.com</p>
</li>
<li>
<a>name</a>
<p>https://www.fujieb.com</p>
</li>
</ul>`;
// 正则
const reg = /<li>.*?<a>(.*?)<\/a>.*?<p>(.*?)<\/p>/sg;
const result = str.matchAll(reg);
// 返回的是可迭代对象,可用扩展运算符展开
console.log(...result);
// 使用for...of...遍历
for(let v of result){
console.log(v);
}
// 打印结果
['<li><a>name</a><p>https://www.fujuhao.com</p>', 'name', 'https://www.fujuhao.com', index: 4, input: '<ul><li><a>name</a><p>https://www.fujuhao.com</p><…a>name</a><p>https://www.fujieb.com</p></li></ul>', groups: undefined]
['<li><a>name</a><p>https://www.fujieb.com</p>', 'name', 'https://www.fujieb.com', index: 54, input: '<ul><li><a>name</a><p>https://www.fujuhao.com</p><…a>name</a><p>https://www.fujieb.com</p></li></ul>', groups: undefined]
ES11新特性 2、类的私有属性
ES11的 私有属性外部不可访问直接;
class Person{
// 公有属性
name;
// 私有属性
#age;
#weight;
// 构造方法
constructor(name, age, weight){
this.name = name;
this.#age = age;
this.#weight = weight;
}
intro(){
console.log(this.name); // fujuhao.com
console.log(this.#age); // 18
console.log(this.#weight); // 90kg
}
}
// 实例化
const girl = new Person("fujuhao.com",18,"90kg");
console.log(girl); // Person {name: 'fujuhao.com', #age: 18, #weight: '90kg'}
// 公有属性的访问
console.log(girl.name); // fujuhao.com
// 私有属性的访问
console.log(girl.age); // undefined
// 报错Private field '#age' must be declared in an enclosing class
// console.log(girl.#age);
girl.intro();
ES11新特性 3、Promise.allSettled
ES11的 Promise.allSettled 获取多个promise执行的结果集;
// 声明两个promise对象
const p1 = new Promise((resolve,reject)=>{
setTimeout(()=>{
resolve("商品数据——1");
},1000);
});
const p2 = new Promise((resolve,reject)=>{
setTimeout(()=>{
reject("失败啦");
},1000);
});
// 调用Promise.allSettled方法
const result = Promise.allSettled([p1,p2]);
console.log(result);
const result1 = Promise.all([p1,p2]);// 注意区别
console.log(result1);
ES11新特性 3、Promise.allSettled 运行结果:
ES11新特性 4、可选链操作符
如果存在则往下走,省略对对象是否传入的层层判断;
// 可选链操作符
// ?.
function main(config){
// 传统写法
// const dbHost = config && config.db && config.db.host;
// 可选链操作符写法
const dbHost = config?.db?.host;
console.log(dbHost); // 192.168.1.100
}
main({
db:{
host:"192.168.1.100",
username:"root"
},
cache:{
host:"192.168.1.200",
username:"admin"
}
});
ES11新特性 5、动态 import 导入
ES11的 动态导入模块,什么时候使用时候导入;
- hello.js:
export function hello(){
alert('Hello');
}
- app.js:
// 传统静态导入
// import * as m1 from "./hello.js";
// 获取元素
const btn = document.getElementById('btn');
// 动态导入
btn.onclick = function(){
import('./hello.js').then(module => {
module.hello();
});
}
- 动态import加载.html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>动态 import </title>
</head>
<body>
<button id="btn">点击</button>
<script src="app.js" type="module"></script> </body>
</html>
ES11新特性 5、动态 import 导入 运行结果:
ES11新特性 6、BigInt
ES11的 BigInt 更大的整数;
// BigInt 大整型
let n = 100n;
console.log(n,typeof(n)); // 100n 'bigint'
// 函数:普通整型转大整型
let m = 123;
console.log(BigInt(m)); // 123n
// 用于更大数值的运算
let max = Number.MAX_SAFE_INTEGER;
console.log(max); // 9007199254740991
console.log(max+1); // 9007199254740992
console.log(max+2); // 9007199254740992 // 出错了
console.log(BigInt(max)); // 9007199254740991n
console.log(BigInt(max)+BigInt(1)); // 9007199254740992n
console.log(BigInt(max)+BigInt(2)); // 9007199254740993n
ES11新特性 7、globalThis 对象
ES11的 globalThis 对象 始终指向全局对象window;
// globalThis 对象 : 始终指向全局对象window
console.log(globalThis);
ES11新特性 7、globalThis 对象 运行结果:
The Posts
- ES11新特性_matchAll_私有属性_allSettled_可选链操作符_动态import导入_BigInt_globalThis对象Mar 24, 2022
- ES10新特性_fromEntries_trimStart_trimEnd_flat_flatMap_descriptionMar 24, 2022
- ES9_正则扩展_正则表达式_命名捕获分组_反向断言_dotAll 模式Mar 23, 2022
- ES8新特性_ECMAScript8_object对象方法扩展Mar 19, 2022
- ES8新特性_ECMAScript8_async_awaitMar 19, 2022
- ES7新特性_ECMAScript7_includes_指数运算符Mar 19, 2022
- ES6新特性_模块化(module)Mar 17, 2022
- ES6新特性_number数值扩展与object对象方法扩展Mar 16, 2022
- ES6新特性_class类声明继承constructor,super,static,set,get详细介绍Mar 12, 2022
- ES6新特性_Map用法和使用场景size,get,set,has,clear,deleteMar 12, 2022
- ES6新特性_集合(set)Mar 10, 2022
- ES6新特性_Promise介绍与基本使用Mar 10, 2022
- ES6新特性_生成器函数Mar 9, 2022
- ES6新特性_迭代器Mar 9, 2022
- ES6新特性_Symbol基本使用Mar 9, 2022
- ES6新特性_扩展运算符Mar 9, 2022
- ES6新特性_rest参数Mar 8, 2022
- ES6新特性_函数参数的默认值Mar 8, 2022
- ES6新特性_箭头函数Mar 8, 2022
- ES6新特性_简化对象的写法Mar 8, 2022